How to configure and use the Python interpreter
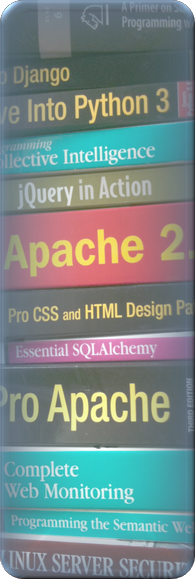
Introduction to the Python interpreter
The interactive prompt
The interactive prompt allows interacting with the Python interpreter from a terminal. Once the interactive prompt has been launched, it is possible to enter valid Python code line by line. A line of the interactive prompt always starts with the characters >>> or ... depending on indentation.
$ python Python 2.6.5 (r265:79063, Apr 16 2010, 13:09:56) [GCC 4.4.3] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> prompt = "guest" >>> if prompt: ... print prompt ... guest >>>
To exit from the Python interactive prompt, we'll hit Ctrl+D under linux ubuntu. Since the interactive prompt plays an important role in the development of Python code, we would customize it for a more efficient use. This is explained in the following paragraph.
The start-up file of the Python interactive prompt
The interactive prompt can be configured with a start up file. When firing it up, the interactive prompt search the environment variable PYTHONSTARTUP and executes the code which is into the file pointed to by this variable. Some Linux distributions provide a default start up script, which is usually located in the home directory; this script is called .pythonstartup. Features, such as words completion by the TAB key, command history, are often required to improve the interactive prompt functionality. These features are based on the module readline, which is therefore required. It is also possible to create a simple start up script as it follows:
import readline import rlcompleter import atexit import os # tab completion readline.parse_and_bind('tab: complete') # history file histfile = os.path.join(os.environ['HOME'], '.pythonhistory') try: readline.read_history_file(histfile) except IOError: pass atexit.register(readline.write_history_file, histfile) del os, histfile, readline, rlcompleter
In a Linux system, the easiest way is to create the start up script in the home directory and call it .pythonstartup. Then we can add a system environment variable PYTHONSTARTUP which would point to the .pythonstartup script. This can be done, for example, by using the .profile (or rather .bashrc), where we can initialize the variable PYTHONSTARTUP by inserting a line like the following one:
export PYTHONSTARTUP=~/.pythonstartup
When the interactive prompt is launched, the script .pythonstartup should be executed and the new features become available. For example, the words completion features by the TAB key is really useful to introspect all attributes of any Python object:
>>> import hashlib >>> hashlib.<tab> hashlib.__class__( hashlib.__loader__ hashlib.__subclasshook__( hashlib.__delattr__( hashlib.__name__ hashlib._hashlib hashlib.__dict__ hashlib.__new__( hashlib.md5( hashlib.__doc__ hashlib.__package__ hashlib.new( hashlib.__file__ hashlib.__py_new( hashlib.sha1( hashlib.__format__( hashlib.__reduce__( hashlib.sha224( hashlib.__get_builtin_constructor( hashlib.__reduce_ex__( hashlib.sha256( hashlib.__getattribute__( hashlib.__repr__( hashlib.sha384( hashlib.__hash__( hashlib.__setattr__( hashlib.sha512( hashlib.__hash_new( hashlib.__sizeof__( hashlib.__init__(
An advanced Python interactive interpreter
We can adapt the start up script .pythonstartup for more automation. In addition, the module code provides the base classes of the interpreter. However there is already an advanced Python interactive interpreter, called IPython, with features such as dynamic introspection of objects, system terminal access from the Python prompt, debugging tools, etc..
Launch the Python interactive interpreter from the system terminal
Launching the Python interactive interpreter from the system terminal (by simply editing python), gives access to a certain number of options:
$ python [-dEiOQsStuvVxX3?] [-c command | -m module-name | script | - ] [args] $ python -V Python 2.6.5
Any arguments following the python command is available from the attribute sys.argv, with for instance the first element available at sys.argv[0]. The most common usage of the Python interpreter is, of course, a simple invocation of the filename of a Python script. In this case, the Python interpreter reads and executes the script from that file:
$ python script.py [args]
When called with the -c command, it executes the instructions data given in place of command. The set of instructions referred to by command may contain multiple lines separated by line breaks, taking into account the necessary indentation spaces. If this option is used, the first element of sys.argv will be -c and the current directory will be added at the beginning of sys.path, allowing modules in that directory to be imported.
$ python -c """ > a=2; b=3 > if a: print a+b""" 5 $
When the Python interpreter is called with -m module-name, the given module is located on the Python modules import paths and is run as a script. The module name given does not contain the .py extension.
$ python -m module [args]
With the -i option we can, all at once, launch a python script, run it, start the Python interactive interpreter and access the attributes of the script. For example, let's consider a Python script file in the current directory, named interpreter.py, containing the following script:
#!/usr/local/bin/python2.7 # -*- coding: utf-8 -*- # # Copyright 2012 RasadaCrea. All rights reserved a_string = 'string' an_integer = 11 an_arg = 'an_arg_of_function' def a_function(an_arg): return an_arg class AClass: pass an_instance = AClass() print '%s - %d - %s - %s' % (a_string, an_integer, a_function(an_arg), an_instance)
Launching this script, from the terminal, with the -i option of the python command, we could get the following experience:
$ python -i interpreter.py string - 11 - an_arg_of_function - <__main__.AClass instance at 0xb738ca0c> >>> vars() {'an_integer': 11, '__builtins__': <module '__builtin__' (built-in)>, 'AClass': <class __main__.AClass at 0xb7382a7c>, '__package__': None, 'an_instance': <__main__.AClass instance at 0xb738ca0c>, 'an_arg': 'an_arg_of_function', '__name__': '__main__', '__doc__': None, 'a_string': 'string', 'a_function': <function a_function at 0xb737da74>} >>> an_integer 11 >>> a_function <function a_function at 0xb737da74> >>> a_function('another argument') 'another argument' >>> an_instance <__main__.AClass instance at 0xb738ca0c>
Individual training and Python learning programming courses by RasadaCrea
All Python programming courses taught by RasadaCrea are individuals and personals. Contact RasadaCrea for more information about creating your personalized Python programming training plan, as well as for prices and organization of the Python learning programs.
RasadaCrea Tweets Social Marketing
Django web individual training courses and easy python learning http://t.co/WUIj03Q2 #solution #training 13 days ago
Learn how to process log files with Python scripts: RasadaCrea learning courses http://t.co/lqCdPEmK #web #training 70 days ago
Apply newsletter marketing with a django based solution by RasadaCrea http://t.co/ZfvvW4YH #solution #training 90 days ago